Java Object-Oriented Programming: Encapsulation, Inheritance, and Polymorphism
- Chris Wong
- Jun 17, 2023
- 4 min read
Welcome to this informative web post, where we'll delve into three of the most fundamental concepts in Java's object-oriented programming paradigm: encapsulation, inheritance, and polymorphism. These principles play a crucial role in structuring and organizing your code, improving its readability, reusability, and maintainability.
In the following sections, we'll explore each concept in detail, providing clear examples and practical use cases. By understanding these key principles, you'll be well-equipped to design robust and efficient Java applications. So, let's embark on this journey and discover the power of encapsulation, inheritance, and polymorphism in Java!
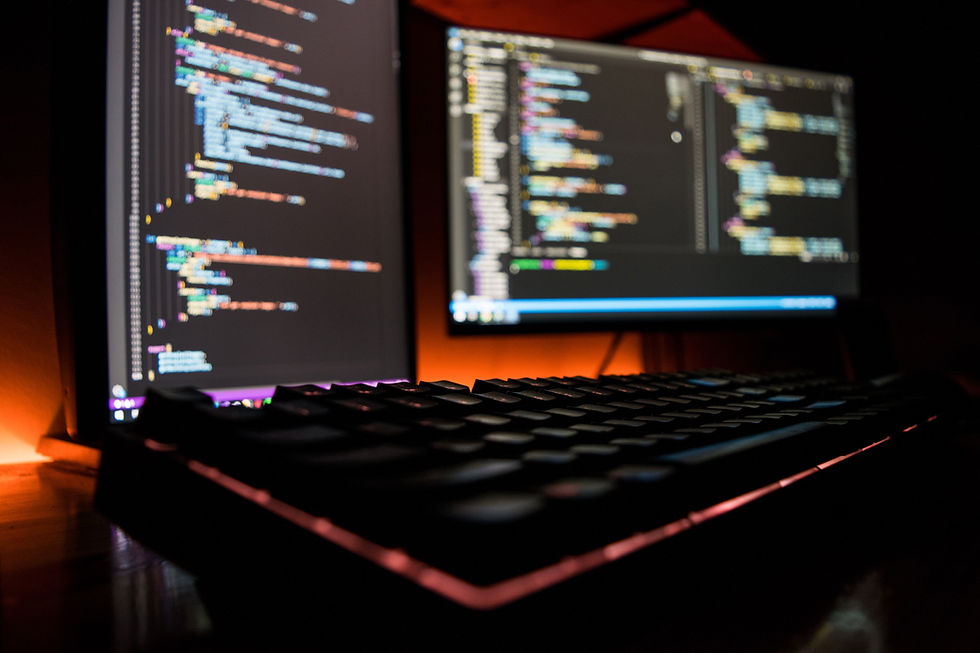
Encapsulation
Encapsulation is like having a toy box where you keep your toys. The toy box helps you to keep all your toys together (data and actions) in one place. You can open the toy box and take out a toy (access data) or put one back (change data), but you can't just throw a toy into the box from across the room (directly access the data).
In programming, encapsulation is like having a "code toy box" that keeps data and actions related to a specific thing, like a car or a person, all together in one place. This "code toy box" is usually called a class.
We use encapsulation to make sure other parts of the code can't mess around with our "code toy box" in a way we don't want them to. We create special ways to interact with the stuff inside the "code toy box", like opening the lid (using methods) to get or change what's inside (attributes).
For example, let's say we have a class called "Bicycle". Inside this class, we keep information about the bike, like its color and the number of gears it has. We also have actions we can do with the bike, like changing gears or ringing the bell. We use encapsulation to make sure that other parts of our code can't directly change the color or number of gears without using the special methods we created. This helps us keep our code organized and easy to understand.
Exercise 1: Bank Account
Create a BankAccount class that demonstrates the concept of encapsulation. The class should have private fields for the account number, account holder's name, and account balance. Provide public methods to access and manipulate these fields.
public class BankAccount {
private String accountNumber;
private String accountHolderName;
private double balance;
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public String getAccountHolderName() {
return accountHolderName;
}
public void setAccountHolderName(String accountHolderName) {
this.accountHolderName = accountHolderName;
}
public double getBalance() {
return balance;
}
public void deposit(double amount) {
if (amount > 0) {
balance += amount;
} else {
System.out.println("Amount should be positive.");
}
}
public void withdraw(double amount) {
if (amount > 0 && amount <= balance) {
balance -= amount;
} else {
System.out.println("Invalid withdrawal amount.");
}
}
}
Polymorphism
Polymorphism is a fundamental concept in object-oriented programming (OOP) that allows objects of different classes to be treated as objects of a common superclass. In Java, polymorphism enables you to write more flexible and reusable code by allowing a single method or variable to work with different data types or objects.
Polymorphism in Java can be achieved through inheritance and interfaces. The key to polymorphism is that a method or variable declared with a superclass type can hold a reference to an object of any of its subclasses.
Inheritance and Polymorphism
Polymorphism and inheritance are not the same, but they are closely related concepts in object-oriented programming (OOP). Let's clarify the differences and relationships between the two:
Inheritance is a mechanism in which one class acquires the properties (fields) and behaviors (methods) of another class. It enables you to create new classes that are built upon existing classes. Inheritance promotes code reusability and organization. The class that is inherited from is called the superclass (or parent/base class), and the class that inherits from the superclass is called the subclass (or derived/child class). In Java, inheritance is achieved using the extends keyword.
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables you to write more flexible and reusable code, as a single method or variable can work with different data types or objects. Polymorphism takes advantage of inheritance and can be achieved through method overriding and interfaces in Java.
While inheritance is focused on creating a hierarchy of related classes, sharing common properties and behaviors, polymorphism is about using these related classes interchangeably through a common interface (in the general sense, not necessarily a Java interface). In other words, inheritance is the foundation for building a class hierarchy, and polymorphism allows you to leverage that hierarchy to write more flexible and reusable code.
Exercise 2: Java Inheritance
In this exercise, you will create a simple class hierarchy to learn about Java inheritance. You will create a base class `Animal` and two derived classes, `Dog` and `Cat`.
1. Create a base class `Animal` with the following properties and methods:
- A private `String` instance variable `name`.
- A constructor that takes a `String` parameter and sets the `name` variable.
- A public `speak()` method that prints "The animal makes a sound.".
- A public `getName()` method that returns the `name` variable.
2. Create a derived class `Dog` that inherits from the `Animal` class:
- Override the `speak()` method to print "The dog barks.".
3. Create another derived class `Cat` that also inherits from the `Animal` class:
- Override the `speak()` method to print "The cat meows.".
4. In the `main()` method of your program:
- Create instances of `Dog` and `Cat`, and call their `speak()` and `getName()` methods.
class Animal {
private String name;
public Animal(String name) {
this.name = name;
}
public void speak() {
System.out.println("The animal makes a sound.");
}
public String getName() {
return name;
}
}
class Dog extends Animal {
public Dog(String name) {
super(name);
}
@Override
public void speak() {
System.out.println("The dog barks.");
}
}
class Cat extends Animal {
public Cat(String name) {
super(name);
}
@Override
public void speak() {
System.out.println("The cat meows.");
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog("Buddy");
Cat cat = new Cat("Whiskers");
dog.speak();
cat.speak();
System.out.println("Dog's name: " + dog.getName());
System.out.println("Cat's name: " + cat.getName());
}
}
Commenti